Last updated on January 31st, 2025 at 09:40 pm
We will also discuss what is recursive algorithm, Recursion Sum Function, Is Palindrome Recursion C, Base Case Recursion, and Recursion Problems.
Table of Contents
1. What Is Recursive Algorithm?
A recursive algorithm is a type of algorithm that solves a problem by breaking it down into smaller sub-problems of the same type. The algorithm solves each sub-problem recursively until it reaches a base case that can be solved directly. The recursive algorithm then combines the solutions to the sub-problems to solve the original problem.
A recursive method that solves a problem by calling a copy of itself to work on a smaller problem is called a recursion step.
A Recursive function performs a task in part by calling itself to perform the subtasks. Recursive code is generally shorter and easier to write than iterative code.
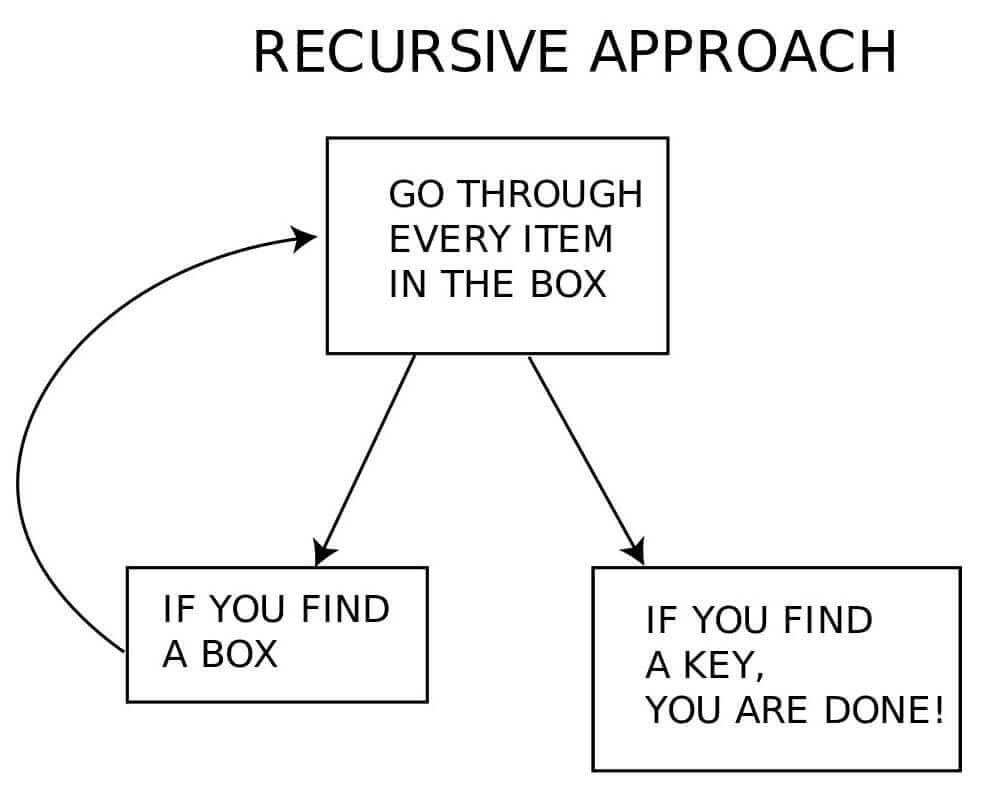
public static long fib (long n) { if (n <= 1) return n; else return fib (n-1) + fib (n-2);
2. How Does Recursion Work?
Recursion works by using a function that calls itself repeatedly until it reaches a base case. The function solves each sub-problem recursively, and the solutions are combined to solve the original problem. The recursive function consists of two main components:
- Base Case Recursion: This is the condition under which the recursion stops. Without a base case, a recursive function would continue to call itself indefinitely, leading to a stack overflow error.
- Recursive Case: The recursive case is the part of the function that calls itself repeatedly until it reaches the base case.
3. Types of Recursion
There are two main types of recursion:
- Direct Recursion: In direct recursion, the function calls itself directly.
- Indirect Recursion: In indirect recursion, the function calls another function that in turn calls the original function.
4. Exploring Recursion Sum Function
The recursion sum function is a simple yet effective example of a recursive algorithm. It calculates the sum of a list of numbers by adding the first number to the sum of the remaining numbers. The base case occurs when the list is empty, returning a sum of zero.
def recursive_sum(n): if n == 0: return 0 else: return n + recursive_sum(n - 1)
5. Is Palindrome Recursion C: A Practical Example
Checking if a string is a palindrome (reads the same forwards and backwards) can be efficiently done using recursion in C. The recursive function compares the first and last characters of the string, then calls itself with the substring that excludes these characters. The base case is reached when the string length is 0 or 1, indicating a palindrome.
#include <stdio.h> #include <string.h> int is_palindrome(char str[], int start, int end) { if (start >= end) return 1; if (str[start] != str[end]) return 0; return is_palindrome(str, start + 1, end - 1); } int main() { char str[] = "madam"; int len = strlen(str); if (is_palindrome(str, 0, len - 1)) printf("%s is a palindrome\n", str); else printf("%s is not a palindrome\n", str); return 0; }
6. Advantages of Recursion
- Divide and Conquer: Solve complex problems by breaking them down into smaller sub-problems.
- Elegant Code: Elegant and concise code.
- Easy to Understand: Make code easier to understand by breaking down complex problems into smaller sub-problems.
7. Common Recursion Problems and Solutions
Some common recursion problems include:
- Stack Overflow: If the recursion depth exceeds the stack size, it can lead to a stack overflow error.
- Performance Issues: Less efficient than their iterative counterparts.
- Complexity: Harder to understand and debug.
8. Examples of Recursive Algorithms
To better understand what is a recursive algorithm, let’s explore some common examples:
- Factorial Calculation: The factorial of a number n (denoted as n!) is the product of all positive integers less than or equal to n. A recursive algorithm for calculating factorials involves multiplying n by the factorial of n-1, with the base case being that the factorial of 0 is 1.
- Fibonacci Sequence: This sequence is another classic example of recursion, where each number is the sum of the two preceding ones. The recursive algorithm for generating Fibonacci numbers involves calling the function with the two previous numbers until reaching the base cases of 0 and 1.
FAQs
1. What is the difference between recursion and iteration?
Recursion involves a function calling itself, while iteration uses loops to repeat a set of instructions.
2. Why use recursion instead of iteration?
Recursion can simplify code and make it more readable, especially for problems that naturally fit a recursive pattern.
3. What are the disadvantages of recursion?
Recursion can lead to stack overflow and may be less efficient than iterative solutions.
4. How do you identify a base case in recursion?
A base case is a condition that stops the recursion, often when the problem is reduced to its simplest form.
5. Can recursion be used to solve dynamic programming problems?
Yes, recursion can be used to solve dynamic programming problems.
6. Is recursion an efficient way to solve problems?
Recursion can be inefficient due to the overhead of function calls, but it can also be an efficient way to solve problems by breaking them down into smaller sub-problems.