Last updated on January 31st, 2025 at 09:37 pm
Here, This article will explore the iterative algorithm definition, explore its applications, and compare it with recursive approaches.
Table of Contents
1. Iterative Algorithm Definition
An iterative algorithm, also known as an iterated algorithm, is a problem-solving process that repeats a specific sequence of instructions until a desired outcome or termination condition is met. It involves breaking down a problem into smaller, manageable steps and executing them in a loop-like manner.
Iteration is a technique in which a function calls repeatedly for a finite number of times.
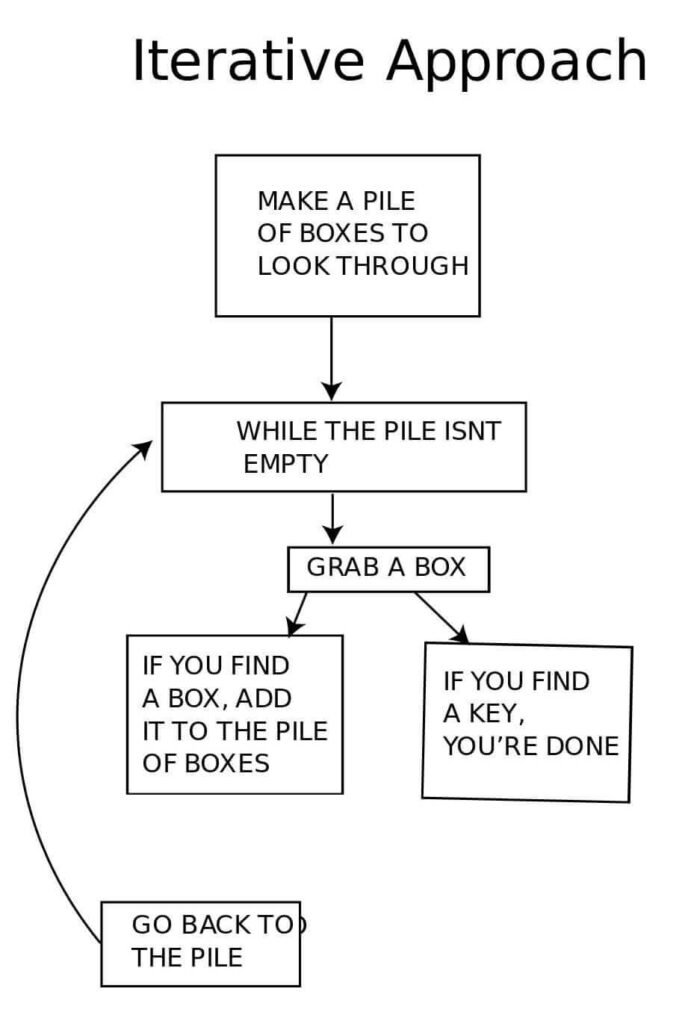
2. How Does an Iterative Algorithm Work?
Here’s a breakdown of the Iterative Algorithm process:
- Initialization: The algorithm starts with an initial state or input.
- Iteration: A set of instructions is repeatedly executed, often updating variables or data structures.
- Termination: The loop continues until a specific condition is satisfied, ensuring the algorithm concludes with a solution.
This approach allows for gradual improvement towards the desired result, making it ideal for various computational tasks.
3. Implementation of Iterative Algorithm
public static long fib (long n) { if ((n == 1) || (n == 2)){ return 1; } else{ long prev = 1, current = 1, next = 0; for (long i=3; i<=n; i++){ next = prev + current; prev = current; current = next; } return next; } }
4. Iterative Binary Search Algorithm
The binary search algorithm is a classic example of an iterative algorithm. It repeatedly divides the search interval in half. If the value of the search key is less than the item in the middle of the interval, the algorithm narrows the interval to the lower half. Otherwise, it narrows it to the upper half. This process continues until the value is found or the interval is empty.
def binary_search(arr, x): low = 0 high = len(arr) - 1 mid = 0 while low <= high: mid = (high + low) // 2 if arr[mid] < x: low = mid + 1 elif arr[mid] > x: high = mid - 1 else: return mid return -1
5. Any Recursive Algorithm Can Be Written Iteratively
Recursive algorithms can often be transformed into iterative ones, offering an alternative approach. This is particularly useful for optimizing stack space and avoiding potential stack overflow issues.
5.1 Converting Factorial Recursive to Iterative
# Recursive Approach def factorial_recursive(n): if n == 0: return 1 return n * factorial_recursive(n-1) # Iterative Version def factorial_iterative(n): result = 1 for i in range(1, n+1): result *= i return result
6. Advantages of Iterative Algorithms
- Use less memory because they do not require additional stack space for each recursive call.
- More efficient in terms of execution time, especially for large inputs.
- Allows for dynamic adjustments and optimizations during runtime.
- Well-suited for dealing with infinite or large data streams.
7. Disadvantages of Iterative Algorithms
- Some problems are naturally recursive and can be more complex to solve iteratively.
- Recursive solutions can be more elegant and easier to read for certain problems.
8. Common Applications of Iterative Algorithm
Iterative algorithms are widely used in various fields, including:
- Computer Networks: Iterative algorithms are used in computer networks to optimize routing and scheduling.
- Data Analysis: Iterative algorithms are used in data analysis to process large datasets and perform complex calculations.
- Machine Learning: Iterative algorithms are used in machine learning to train models and optimize parameters.
FAQs
1. How does an iterative algorithm differ from a recursive algorithm?
Iterative algorithms use loops, while recursive algorithms use self-calling functions. Iterative algorithms typically use less memory and can be more efficient.
2. Can any recursive algorithm be written iteratively?
Yes, any recursive algorithm can be written iteratively, although it may increase complexity.
3. What are some examples of iterative algorithms?
Iterative algorithms are widely used in data analysis, machine learning, and computer networks. Examples include iterative binary search, bubble sort, and iterative deepening search.
4. Is an iterative algorithm always better than a recursive algorithm?
Not always. While iterative algorithms are often more efficient, recursive algorithms can be more intuitive and easier to understand for certain problems.
5. How do iterative algorithms handle complex problems with multiple variables?
Iterative algorithms can manage complex problems by updating multiple variables within the loop. Each iteration refines the solution based on the current state, gradually converging towards the desired outcome.