Last updated on March 7th, 2025 at 09:39 pm
Here, we see a Rotate Image LeetCode Solution. This Leetcode problem is solved using different approaches in many programming languages, such as C++, Java, JavaScript, Python, etc.
List of all LeetCode Solution
Topics
Array, Math, Matrix
Companies
Amazon, Apple, Microsoft
Level of Question
Medium

Rotate Image LeetCode Solution
Table of Contents
1. Problem Statement
You are given an n x n 2D matrix representing an image, rotate the image by 90 degrees (clockwise).
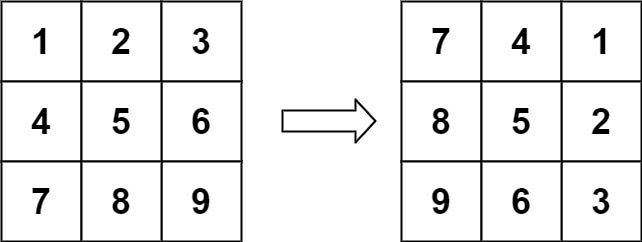
Example 1: (fig-1)
Input: matrix = [[1,2,3],[4,5,6],[7,8,9]]
Output: [[7,4,1],[8,5,2],[9,6,3]]
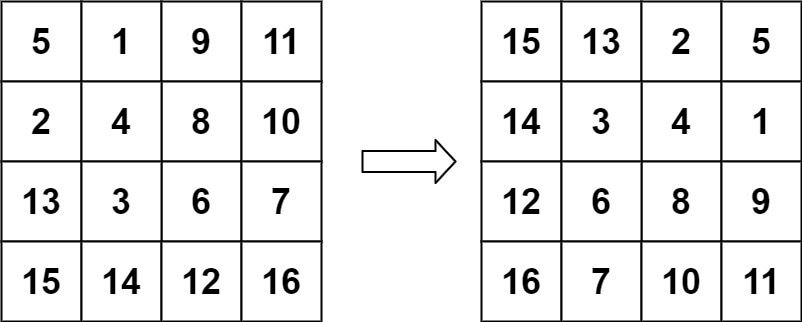
Example 2: (fig-2)
Input: matrix = [[5,1,9,11],[2,4,8,10],[13,3,6,7],[15,14,12,16]]
Output: [[15,13,2,5],[14,3,4,1],[12,6,8,9],[16,7,10,11]]
2. Coding Pattern Used in Solution
The coding pattern used in all the provided implementations is “Matrix Manipulation”. This pattern involves performing operations directly on a 2D matrix, such as transposing, rotating, flipping, or other transformations.
3. Code Implementation in Different Languages
3.1 Rotate Image C++
class Solution { public: void rotate(vector<vector<int>>& matrix) { int n = matrix.size(); for(int i=0; i<n; i++) { for(int j=i; j<n; j++) { int temp = matrix[i][j]; matrix[i][j] = matrix[j][i]; matrix[j][i] = temp; } } for(int i=0; i<n; i++) { reverse(matrix[i].begin(), matrix[i].end()); } } };
3.2 Rotate Image Java
class Solution { public void rotate(int[][] matrix) { for(int i = 0; i<matrix.length; i++){ for(int j = i; j<matrix[0].length; j++){ int temp = 0; temp = matrix[i][j]; matrix[i][j] = matrix[j][i]; matrix[j][i] = temp; } } for(int i =0 ; i<matrix.length; i++){ for(int j = 0; j<matrix.length/2; j++){ int temp = 0; temp = matrix[i][j]; matrix[i][j] = matrix[i][matrix.length-1-j]; matrix[i][matrix.length-1-j] = temp; } } } }
3.3 Rotate Image JavaScript
var rotate = function(matrix) { let n = matrix.length, depth = ~~(n / 2) for (let i = 0; i < depth; i++) { let len = n - 2 * i - 1, opp = n - 1 - i for (let j = 0; j < len; j++) { let temp = matrix[i][i+j] matrix[i][i+j] = matrix[opp-j][i] matrix[opp-j][i] = matrix[opp][opp-j] matrix[opp][opp-j] = matrix[i+j][opp] matrix[i+j][opp] = temp } } };
3.4 Rotate Image Python
class Solution(object): def rotate(self, matrix): l = 0 r = len(matrix) -1 while l < r: matrix[l], matrix[r] = matrix[r], matrix[l] l += 1 r -= 1 for i in range(len(matrix)): for j in range(i): matrix[i][j], matrix[j][i] = matrix[j][i], matrix[i][j]
4. Time and Space Complexity
Time Complexity | Space Complexity | |
C++ | O(n2) | O(1) |
Java | O(n2) | O(1) |
JavaScript | O(n2) | O(1) |
Python | O(n2) | O(1) |
- The code uses the Matrix Manipulation pattern to rotate the matrix in-place.
- The time complexity for all implementations is
O(n2)
because every element in the matrix is processed once. - The space complexity is
O(1)
because the rotation is performed in-place without using additional data structures.