Last updated on March 9th, 2025 at 12:13 am
Here, we see a Delete Node in a Linked List LeetCode Solution. This Leetcode problem is solved using different approaches in many programming languages, such as C++, Java, JavaScript, Python, etc.
List of all LeetCode Solution
Topics
Linked List
Companies
Adobe, Apple, Microsoft
Level of Question
Medium

Delete Node in a Linked List LeetCode Solution
Table of Contents
1. Problem Statement
There is a singly-linked list head and we want to delete a node in it.
You are given the node to be deleted node. You will not be given access to the first node of head.
All the values of the linked list are unique, and it is guaranteed that the given node is not the last node in the linked list.
Delete the given node. Note that by deleting the node, we do not mean removing it from memory. We mean:
- The value of the given node should not exist in the linked list.
- The number of nodes in the linked list should decrease by one.
- All the values before node should be in the same order.
- All the values after node should be in the same order.
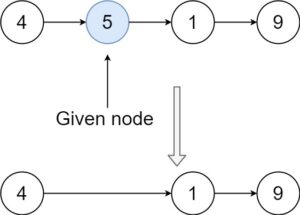
Example 1: (fig-1) Input: head = [4,5,1,9], node = 5 Output: [4,1,9] Explanation: You are given the second node with value 5, the linked list should become 4 -> 1 -> 9 after calling your function.

Example 2: (fig-2) Input: head = [4,5,1,9], node = 1 Output: [4,5,9] Explanation: You are given the third node with value 1, the linked list should become 4 -> 5 -> 9 after calling your function.
2. Coding Pattern Used in Solution
The coding pattern used here is “In-place Node Manipulation in a Linked List”. It involves modifying the linked list directly without creating new nodes or using additional data structures.
3. Code Implementation in Different Languages
3.1 Delete Node in a Linked List C++
class Solution { public: void deleteNode(ListNode* node) { node->val = node->next->val; node->next = node->next->next; } };
3.2 Delete Node in a Linked List Java
class Solution { public void deleteNode(ListNode node) { if(node != null && node.next != null) { node.val = node.next.val; node.next = node.next.next; } } }
3.3 Delete Node in a Linked List JavaScript
var deleteNode = function(node) { node = Object.assign(node, node.next); node = null; return; };
3.4 Delete Node in a Linked List Python
class Solution(object): def deleteNode(self, node): node.val=node.next.val node.next=node.next.next
4. Time and Space Complexity
Time Complexity | Space Complexity | |
C++ | O(1) | O(1) |
Java | O(1) | O(1) |
JavaScript | O(1) | O(1) |
Python | O(1) | O(1) |
- The code uses an in-place modification approach to delete a node in a singly linked list without access to the head.
- The time and space complexity for all implementations is O(1).
- The JavaScript implementation is incorrect and needs to be fixed to align with the logic of the other implementations.