Last updated on January 31st, 2025 at 09:16 pm
Here, This article explores the analysis of algorithms, the design and analysis of algorithms, and asymptotic analysis.
Table of Contents
1. What is the Analysis of Algorithms?
If a problem has more than one solution, the technique used to decide which solution is best is known as algorithm analysis.
Analysis of algorithms refers to the task of determining how much computing time and storage space an algorithms requires. It helps us to determine which algorithm is most efficient in terms of time and space consumed.
Analysis is done on two parameters: time complexity and space complexity.
- Time Complexity – It means the time taken by an algorithm to solve a problem.
- Space Complexity means memory space required by an algorithm to solve a problem.
It can be done in two ways:
- Priori Analysis – analysis is done before the execution. It is independent of CPU, OS, and system architecture and it provides uniform estimated values.
- Posteriori Analysis – analysis is done after the execution. It is dependent on system architecture, CPU, OS, etc. It provides non-uniform exact values.
2. Introduction to the Design and Analysis of Algorithms
Design and Analysis of Algorithms involves two main components:
- Designing algorithms to solve specific problems
- Analyzing the algorithm’s efficiency and effectiveness.
This process helps in determining the best possible solution for a given problem, considering factors like time complexity and space complexity.
2.1. Steps in the Design and Analysis of Algorithms
- Problem Definition
- Algorithm Design
- Algorithm Analysis
- Implementation
- Testing and Validation
3. Best Worst and Average case
To analyze the given algorithms, we need to know with which inputs the algorithms take less time (performing well) and with which inputs the algorithms take a long time.
We have three cases to analyze an algorithm – Best, Worst, and Average Case.
3.1 Worst Case
In the worst-case analysis, we calculate the upper bound on the running time of an algorithm. This case causes the maximum number of operations to be executed.
3.2 Average Case
In average-case analysis, we take all possible inputs and calculate computing time for all of its inputs. Sum all the calculated values and divide the sum by the total number of inputs.
3.3 Best Case
In the best-case analysis, we calculate the lower bound on the running time of an algorithm. This case causes the minimum number of operations to be executed.
4. How to Compare Two Algorithms?
To compare algorithms, three steps are :
- Execution times? execution times are specific to a particular computer.
- Number of statements executed? the number of statements varies with the programming language as well as the style of the individual programmer.
- Ideal solution? running time of a given algorithm as a function of the input size n (i.e., f(n))
5. Algorithm Complexity
The efficiency of an algorithm depends upon the time and space used by the algorithm.
Space Complexity – How much memory is used?
Time Complexity – How many primitive operations are executed?
5.1 What is Time Complexity?
Time Complexity of an algorithm represents the amount of time required by the algorithm to solve a problem.
Computational Time is T(n) = c*n where c is the time taken to add 2 bits and n is the number of steps.
5.2 What is Space Complexity?
Space Complexity of an algorithm represents the amount of memory space required by the algorithm in its life cycle.
Space complexity S(P) of any algorithm P is S(P) = C + SP(I) where C is the fixed part and S(I) is the variable part which depends on instance characteristics I.
6. Algorithm Complexity of Common Operations
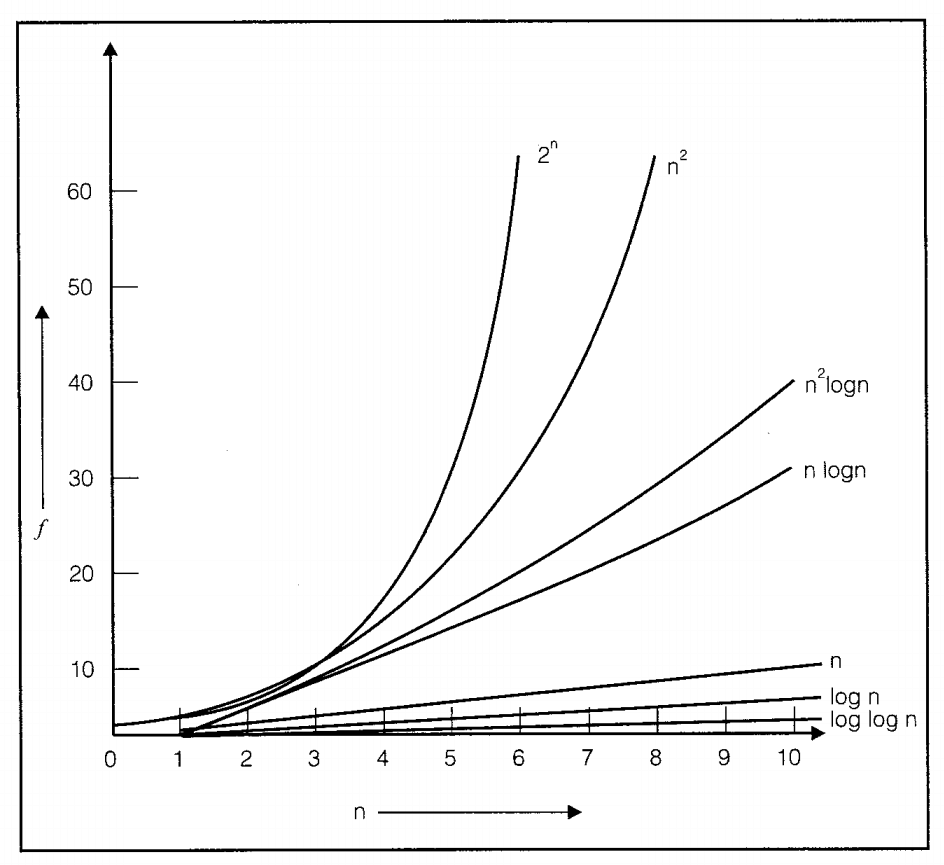
Complexity | Operation |
---|---|
O(1) | Running a statement |
O(1) | Value look-up on an array, object, variable |
O(log n) | A loop that cuts the problem in half every iteration |
O(n) | Looping through the values of an array |
O(n2) | Double nested loops |
7. What is Asymptotic Analysis?
Asymptotic Analysis can be done by actually implementing both algorithms and running them on the same computer with different input values. Then the comparison is made in terms of time.
An algorithm that takes less time is considered better. But there are so many problems with this approach:
- It might be possible that for certain inputs first algorithm performs better than the second and for another set of inputs, the second algorithm performs better. So, the decision of choosing the best among them becomes difficult.
- With the change in the operating machine, the performance of the algorithm varies. On one machine first algorithm can work better and on another machine, the second works better. This is another problem associated with this approach
The above issues can be handled using asymptotic analysis techniques for analyzing algorithms.
In asymptotic analysis, analysis of the algorithm is done in terms of input size. Actual running time is not computed, variation of time with input size is calculated.
FAQs
1. What is the difference between algorithm design and algorithm analysis?
Algorithm design involves creating a step-by-step procedure to solve a problem. Algorithm analysis, on the other hand, involves studying the algorithm’s performance, particularly its time and space complexity.
2. What is the purpose of algorithm analysis?
Algorithm analysis helps in understanding the efficiency and effectiveness of an algorithm, allowing developers to choose the best solution for a given problem.
3. How does asymptotic analysis differ from empirical analysis?
Asymptotic analysis focuses on the theoretical growth rate of an algorithm, while Empirical analysis involves practical testing to observe actual performance.
4. Why is the Design and Analysis of Algorithms important in computer science?
Design and Analysis of Algorithms is crucial because it ensures that software solutions are efficient, scalable, and optimized, which is essential for handling complex computational tasks.
5. What are some common techniques used in algorithm design?
Common techniques include divide and conquer, dynamic programming, greedy algorithms, and backtracking.
6. What is the time complexity of an algorithm?
The time complexity of an algorithm refers to the amount of time it takes to complete as a function of the input size.
7. What is the space complexity of an algorithm?
The space complexity of an algorithm refers to the amount of memory it uses as a function of the input size.
8. What is the difference between a greedy algorithm and a dynamic programming algorithm?
A greedy algorithm makes the locally optimal choice at each step, hoping to find a global optimum solution, while a dynamic programming algorithm breaks down a problem into smaller sub-problems and solves each sub-problem only once.