Last updated on January 31st, 2025 at 08:48 pm
This article will explore the insertion sort, how it works, and Insertion Sort Pseudocode Count Steps comparisons.
Table of Contents
1. What is the Insertion Sort Algorithm?
Insertion Sort is a simple and efficient comparison sort. It is in-place Sorting. The Insertion Sort inserts each element into its proper position. It chooses one element, inserts it into its position, and shifts the rest of the list by one location. This process is repeated until no input element remains.
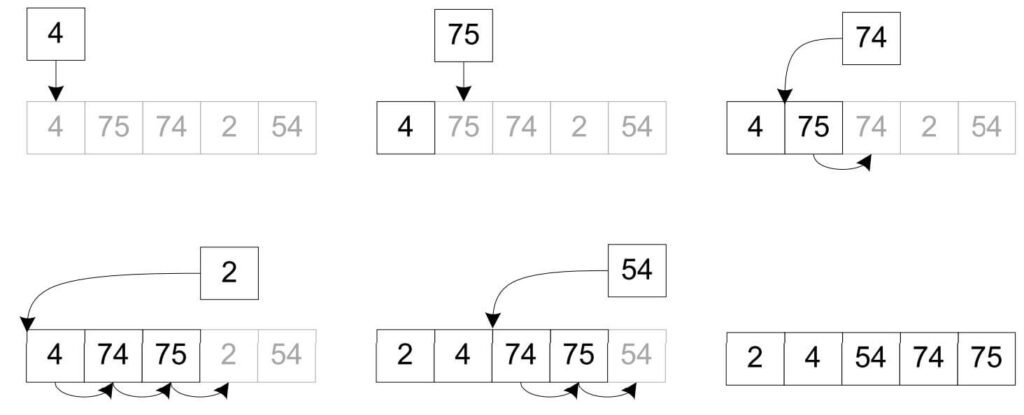
2. How Does Insertion Sort Algorithm Work?
The insertion sort algorithm works by iterating through the array one element at a time, inserting each element into its correct position within the sorted region. The algorithm consists of the following steps:
- Start with the second element: Assume the first element is already sorted.
- Compare the current element with the elements in the sorted portion.
- Shift elements in the sorted portion to the right to make space for the current element.
- Insert the current element into its correct position.
- Repeat the process for all elements in the array.
3. Insertion Sort Pseudocode Count Steps
3.1 Insertion Sort Pseudocode
for i = 1 to length(A) - 1 key = A[i] j = i - 1 while j >= 0 and A[j] > key A[j + 1] = A[j] j = j - 1 A[j + 1] = key
3.2 Counting Steps and Comparisons
To count the steps in insertion sort, you need to consider the number of comparisons and shifts. The worst-case scenario occurs when the array is sorted in reverse order, leading to the maximum number of comparisons and shifts.
- Comparisons: In the worst case, the number of comparisons is approximately n(n−1)/2.
- Shifts: Similarly, the number of shifts is also approximately n(n−1)/2.
4. Recurrence Relation of Insertion Sort
The Recurrence Relation for Insertion Sort is
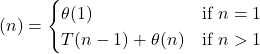
5. Implementing Insertion Sort in CPP
Here’s a simple implementation of insertion sort in C++ (CPP):
#include <iostream> using namespace std; void insertionSort(int arr[], int n) { for (int i = 1; i < n; i++) { int key = arr[i]; int j = i - 1; while (j >= 0 && arr[j] > key) { arr[j + 1] = arr[j]; j = j - 1; } arr[j + 1] = key; } } int main() { int arr[] = {12, 11, 13, 5, 6}; int n = sizeof(arr) / sizeof(arr[0]); insertionSort(arr, n); for (int i = 0; i < n; i++) cout << arr[i] << " "; return 0; }
6. How to Count Comparisons in Insertion Sort
Counting comparisons in insertion sort involves tracking each time an element is compared to another. This can be done by adding a counter within the while loop of the pseudocode. Each time the condition A[j] > key is checked, increment the counter.
comparisons = 0 for i = 1 to n-1 key = arr[i] j = i-1 while j >= 0 and arr[j] > key comparisons = comparisons + 1 arr[j+1] = arr[j] j = j-1 arr[j+1] = key
7. Is Insertion Sort Recursive?
No, the insertion sort algorithm is not recursive. It uses a simple iterative approach to sort the array, making it efficient and easy to implement.
8. Advantages of Insertion Sort
- Simple implementation and easy to understand.
- Efficient for small datasets or nearly sorted data.
- In-place sorting algorithm, meaning it doesn’t require additional memory.
9. Disadvantages of Insertion Sort
- Inefficient for large data sets. Its average and worst-case time complexity is O(n2), where n is the number of elements.
- Not suitable for data sets with a large number of inversions.
10. Time and Space Complexity of Insertion Sort
Time Complexity | |
Worst Case | O(n2) |
Best Case | O(n) |
Average Case | O(n2) |
Space Complexity | |
Worst Case | O(n2) total O(1) auxiliary |
FAQs
1. Why is insertion sort preferred for small datasets?
Insertion sort is preferred for small datasets because it is simple to implement and has low overhead. It is also adaptive, meaning it performs better on partially sorted data.
2. Can insertion sort be used for linked lists?
Yes, insertion sort can be adapted for linked lists. The process is similar, but instead of shifting elements, you adjust pointers.
3. How does insertion sort compare to other sorting algorithms?
Insertion sort is less efficient on large datasets compared to algorithms like quicksort or mergesort. However, it is more efficient than bubble sort and selection sort for small datasets.
4. Is insertion sort a stable sorting algorithm?
Yes, the insertion sort algorithm is a stable sorting algorithm, meaning that it preserves the order of equal elements.
5. Can Insertion Sort be used for large datasets?
While Insertion Sort is simple and efficient for small or nearly sorted lists, it may not be the best choice for large datasets due to its quadratic time complexity. Other sorting algorithms like Merge Sort or Quick Sort are generally preferred for larger data.
6. How does Insertion Sort handle duplicate values?
Insertion Sort handles duplicates efficiently. When a duplicate is encountered, it is inserted next to the existing value, maintaining the relative order of equal elements.
7. What is the time complexity of insertion sort?
The time complexity of insertion sort is O(n^2) in the worst and average cases. However, it performs well on small or nearly sorted datasets.