Last updated on February 4th, 2025 at 12:25 am
Here, we see a Convert BST to Greater Tree LeetCode Solution. This Leetcode problem is solved using different approaches in many programming languages, such as C++, Java, JavaScript, Python, etc.
List of all LeetCode Solution
Topics
Tree
Companies
Amazon
Level of Question
Medium

Convert BST to Greater Tree LeetCode Solution
Table of Contents
1. Problem Statement
Given the root
of a Binary Search Tree (BST), convert it to a Greater Tree such that every key of the original BST is changed to the original key plus the sum of all keys greater than the original key in BST.
As a reminder, a binary search tree is a tree that satisfies these constraints:
- The left subtree of a node contains only nodes with keys less than the node’s key.
- The right subtree of a node contains only nodes with keys greater than the node’s key.
- Both the left and right subtrees must also be binary search trees.
Example 1:
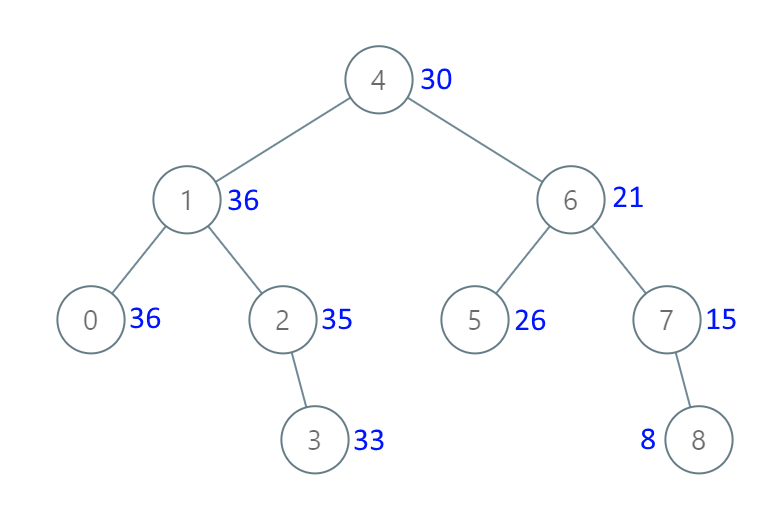
Input: root = [4,1,6,0,2,5,7,null,null,null,3,null,null,null,8]
Output: [30,36,21,36,35,26,15,null,null,null,33,null,null,null,8]
Example 2:
Input: root = [0,null,1]
Output: [1,null,1]
2. Coding Pattern Used in Solution
The coding pattern used in the provided code is “Tree Depth First Search (DFS)”. Specifically, it uses a Reverse Inorder Traversal (right -> root -> left) to traverse the binary search tree (BST). This traversal ensures that nodes are visited in descending order of their values, which is essential for converting the BST into a Greater Tree.
3. Code Implementation in Different Languages
3.1 Convert BST to Greater Tree C++
class Solution { public: TreeNode* convertBST(TreeNode* root) { int num = 0; solve(root, num); return root; } void solve(TreeNode *root, int &num) { if(root == NULL) return; solve(root->right, num); root->val = num + root->val; num = root->val; solve(root->left, num); } };
3.2 Convert BST to Greater Tree Java
class Solution { int sum = 0; public TreeNode convertBST(TreeNode root) { if(root==null){ return root; } reverseInorder(root); return root; } private void reverseInorder(TreeNode root){ if(root==null){ return; } reverseInorder(root.right); root.val = root.val + sum; sum = root.val; reverseInorder(root.left); return; } }
3.3 Convert BST to Greater Tree JavaScript
var convertBST = function(root) { let sum = 0, prev = 0; function rec(root){ if(root){ rec(root.right); sum += root.val; root.val += prev; prev = sum; rec(root.left); } } rec(root); return root; }
3.4 Convert BST to Greater Tree Python
class Solution(object): def convertBST(self, root): def dfs(node): if node: dfs(node.right) node.val += dfs.greater_sum dfs.greater_sum = node.val dfs(node.left) dfs.greater_sum = 0 dfs(root) return root
4. Time and Space Complexity
Time Complexity | Space Complexity | |
C++ | O(n) | O(h) |
Java | O(n) | O(h) |
JavaScript | O(n) | O(h) |
Python | O(n) | O(h) |
where, h is the height of the tree.